pytest是一个单元测试框架,可以帮助写出更优质的程序。flask, werkzeug,gunicorn 等项目中都使用它,本文一起学习如何使用pytest这个测试框架。
原创不易,欢迎加下面的微信和我互动交流,一起进阶:
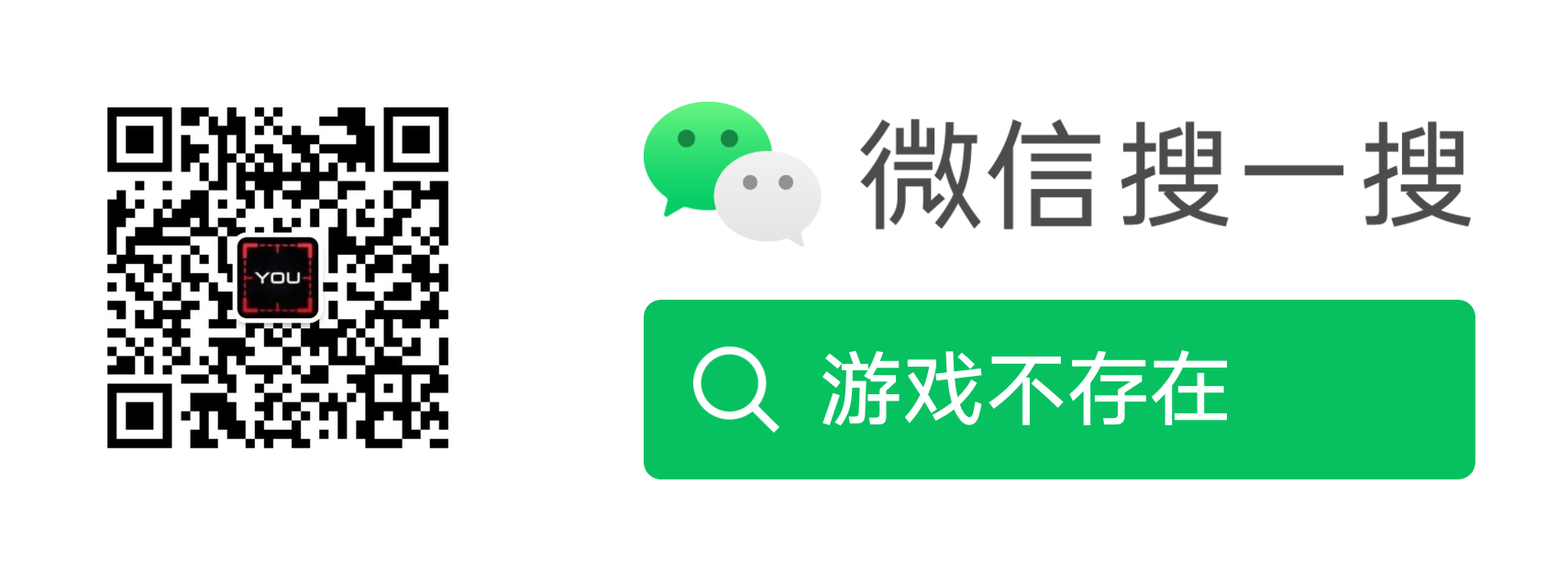
入门用例
简易用例
编写一个简单的示例 test_sample.py
:
1
2
3
4
5
6
|
# cat test_sample.py
def inc(x):
return x + 1
def test_answer():
assert inc(3) == 5
|
示例中定义的两个方法,都非常简单。test_answer
对目标函数 inc
进行断言。使用 pytest test_sample.py
运行测试用例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
# pytest test_sample.py
========================================================================================================= test session starts ==========================================================================================================
platform darwin -- Python 3.7.5, pytest-6.0.2, py-1.9.0, pluggy-0.13.1
rootdir: /Users/yoo/codes/ft6work/devops-py/tests
plugins: parallel-0.1.0, xdist-2.1.0, forked-1.3.0
collected 1 item
test_sample.py F [100%]
=============================================================================================================== FAILURES ===============================================================================================================
_____________________________________________________________________________________________________________ test_answer ______________________________________________________________________________________________________________
def test_answer():
print("test_answer")
> assert inc(3) == 5
E assert 4 == 5
E + where 4 = inc(3)
test_sample.py:7: AssertionError
--------------------------------------------------------------------------------------------------------- Captured stdout call ---------------------------------------------------------------------------------------------------------
test_answer
======================================================================================================= short test summary info ========================================================================================================
FAILED test_sample.py::test_answer - assert 4 == 5
========================================================================================================== 1 failed in 0.04s ===========================================================================================================
|
如果还没有安装pytest,可以使用 pip install pytest
安装。
测试结果显示,运行了一个测试用例,结果是红色,表示失败。错误信息显示,在代码的第7行抛出AssertionError。可以更改一下代码,让测试用例绿色通过。
这个测试用例涉及pytest的3个简单的规则:
- 测试模块以 test_ 前缀命名
- 测试用例(函数)同样以 test_ 前缀命名
- 结果判断使用 assert 断言即可
异常处理
pytest支持异常的捕获, 使用 with + pytest.raises 捕获目标函数的异常:
1
2
3
4
5
6
7
8
9
10
|
# cat test_exception.py
import pytest
def f():
raise SystemExit(1)
def test_mytest():
with pytest.raises(SystemExit):
f()
|
测试类
pytest支持测试类,测试类可以用来做测试用例分组:
1
2
3
4
5
6
7
8
9
|
# cat test_class.py
class TestClass:
def test_one(self):
x = "this"
assert "h" in x
def test_two(self):
x = "hello"
assert hasattr(x, "check")
|
- 类使用 Test 前缀, 并且测试类不需要额外的继承。
自动测试
我们的目录下有下面3个测试模块:
1
2
3
4
|
# ll
-rw-r--r-- 1 yoo staff 168B Jul 22 21:32 test_class.py
-rw-r--r-- 1 yoo staff 117B Jul 22 21:30 test_exception.py
-rw-r--r-- 1 yoo staff 73B Jul 22 21:09 test_sample.py
|
运行pytest
-不带额外参数- 可以自动测试3个模块,非常方便:
1
2
3
4
5
6
7
8
9
10
|
# pytest
============================================================================================== test session starts ===============================================================================================
platform darwin -- Python 3.8.5, pytest-6.2.2, py-1.10.0, pluggy-0.13.1
rootdir: /Users/yoo/work/yuanmahui/python/ch22-pytest
collected 4 items
test_class.py .. [ 50%]
test_exception.py . [ 75%]
test_sample.py . [100%]
=============================================================================================== 4 passed in 0.02s ================================================================================================
|
进阶技巧
了解pytest的基本使用方法后,我们再学习一些进阶技巧,可以帮助我们更好的编写测试用例。
parametrize
parametrize可以减少测试用例的编写。比如下面的测试用例:
1
2
3
4
|
def test_eval():
assert eval("3+5") == 8
assert eval("'2'+'4'") == "24"
assert eval("6*9") == 54
|
对eval的测试条件很多,再增加用例就需要调整函数。这样代码不简洁,也不容易维护。使用 parametrize 可以优化这个问题:
1
2
3
4
5
6
7
|
@pytest.mark.parametrize("test_input,expected", [
("3+5", 8),
("'2'+'4'", "24"),
("6*9", 54)
])
def test_eval_1(test_input, expected):
assert eval(test_input) == expected
|
调整测试函数的参数为输入和期望,然后在parametrize填写参数值,运行时候会自动进行函数参数赋值。这样再增加测试条件,不需要改动test_eval_1的函数体, 增加条件数组即可。
mark
mark是一个标签,可以通过下面方式配置:
1
2
3
4
5
6
|
@pytest.mark.slow
def test_mark():
print("test mark")
# 模拟运行很耗时的测试用例
time.sleep(10)
assert 5 == 5
|
然后目录下增加 pytest.ini
文件,对pytest进行配置:
1
2
3
4
|
# cat pytest.ini
[pytest]
markers =
slow: marks tests as slow (deselect with '-m "not slow"')
|
使用下面命令可以跳过标记的函数,加快测试速度:
1
|
pytest test_sample.py -m "not slow"
|
也可以仅仅运行标记的函数
fixture
fixture可以提供类似初始化和mock功能, 下面是一个完整示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
import pytest
# Arrange
@pytest.fixture
def first_entry():
return "a"
# Arrange
@pytest.fixture
def second_entry():
return 2
# Arrange
@pytest.fixture
def order(first_entry, second_entry):
return [first_entry, second_entry]
# Arrange
@pytest.fixture
def expected_list():
return ["a", 2, 3.0]
def test_string(order, expected_list):
# Act
order.append(3.0)
# Assert
assert order == expected_list
|
可以看到test_string的order参数和expected_list都是前面使用pytest.fixture
装饰器标记的函数结果。fixture还可以嵌套,order嵌套了first_entry和second_entry。
知道fixture如何使用后,可能还有疑问,这样写有什么用?比如测试数据库写入的用例, 需要一个数据库连接参数:
1
2
3
4
|
def test_database_insert_record(database_connection):
if database_connection:
print("Insertion Successful. ", database_connection)
...
|
使用fixture就可以解决这个问题,在测试用例目录编写 conftest.py
:
1
2
3
4
5
|
# cat conftest.py
@pytest.fixture
def database_connection():
# mock...
...
|
plugin&&hook
可以编写pytest的插件plugin和钩子hook对pytest进行扩展。
先创建一个a目录,然后在a目录中创建 conftest.py
和 test-sub.py
:
1
2
3
4
5
6
7
8
9
10
|
# cat a/conftest.py
def pytest_runtest_setup(item):
# called for running each test in 'a' directory
print("setting up", item)
# cat a/test_sub.py
def test_sub():
pass
|
使用 pytest a/test_sub.py --capture=no
会加载我们编写的插件和钩子,在console中可以看到下面字样:
1
2
|
...
a/test_sub.py setting up <Function test_sub>
|
- 使用系统钩子,以 pytest_ 为前缀,名为 runtest_setup 。
使用pytest_runtest_setup可以实现测试框架中的setup类似功能。
小结
pytest是一个简单易用的测试用例,在flask, werkzeug,gunicorn等项目中使用。使用方法如下:
- 测试目录一般使用 tests 命名和src同层级
- 测试模块使用 test_ 前缀
- 测试类使用 Test 前缀,不需要继承其它父类
- 测试用例也使用 test_ 前缀
- 可以使用parametrize进行参数化处理
- 可以使用mark给测试用例加标签
- 可以使用fixture模拟测试条件
- 使用pytest.ini文件对pytest进行配置
- 可以编写插件和hoo对pytest扩展
pytest还有很多用法,下次我们继续学习。
参考链接